Refactoring
Code refactoring is the process of changing the code without modifying the existing functionality of your application. By refactoring your code you can:
- Improve internal quality of your application
- Improve performance and extensibility
- Improve code readability and maintainability
- Simplify code structure
Finding Symbols
To find the use of a specific symbol in your Qt C++ or Qt Quick project:
- In the editor, place the cursor on the symbol, and select:
- Tools > C++ > Find Usages
- Tools > QML > Find Usages
- Ctrl+Shift+U
Qt Creator looks for the symbol in the following locations:
- Files listed as a part of the project
- Files directly used by the project files (for example, generated files)
- Header files of used frameworks and libraries
Note: You can also select Edit > Find/Replace > Advanced Find > C++ Symbols to search for classes, methods, enums, and declarations either from files listed as part of the project or from all files that are used by the code, such as include files.
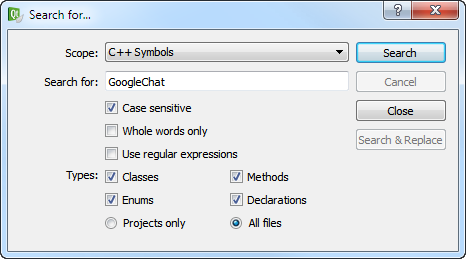
- The Search Results pane opens and shows the location and number of instances of the symbol in the current project.
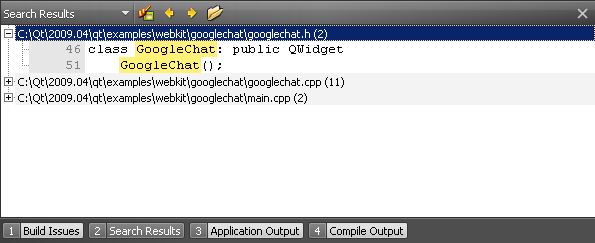
You can browse the search results in the following ways:
- To go directly to an instance, double-click the instance in the Search Results pane.
- To move between instances, click
and in the Search Results pane.
- To expand and collapse the list of all instances, click
.
- To clear the search results, click
.
Renaming Symbols
The functions available for renaming symbols depend on whether you are writing C++ or QML code. For QML, you can only rename IDs.
To rename a specific symbol in a Qt project:
- In the editor, place the cursor on the symbol you would like to change and select Tools > C++ > Rename Symbol Under Cursor or press Ctrl+Shift+R.
The Search Results pane opens and shows the location and number of instances of the symbol in the current project.
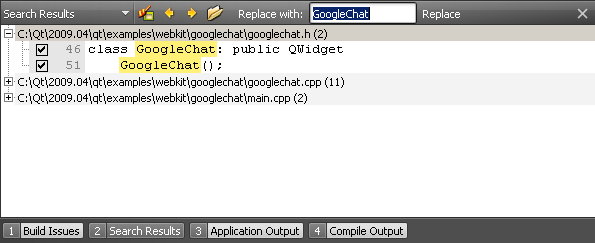
- To replace all selected instances, enter the name of the new symbol in the Replace with text box and click Replace.
To omit an instance, uncheck the check-box next to the instance.
Note: This action replaces all selected instances of the symbol in all files listed in the Search Results pane. You cannot undo this action.
Note: Renaming local symbols does not open the Search Results pane. The instances of the symbol are highlighted in code and you can edit the symbol. All instances of the local symbol are changed as you type.
To rename an ID in a Qt Quick project:
- Right-click an ID in the QML code and select Rename id.
- In the Rename id field, enter the new ID.
Applying Refactoring Actions
Qt Creator allows you to quickly and conveniently apply actions to refactor your code by selecting them in a context menu. The actions available depend on the position of the cursor in the code editor and on whether you are writing C++ or QML code.
To apply refactoring actions to C++ code, right-click an operand, conditional statement, string, or name to open a context menu. In QML code, click an element ID or name.
In the context menu, select Refactoring and then select a refactoring action.
You can also press Alt+Enter to open a context menu that contains refactoring actions available in the current cursor position.
Refactoring C++ Code
You can apply the following types of refactoring actions to C++ code:
- Change binary operands
- Simplify if and while conditions (for example, move declarations out of if conditions)
- Modify strings (for example, set the encoding for a string to Latin-1, mark strings translatable, and convert symbol names to camel case)
- Create variable declarations
- Create method declarations and definitions
The following table summarizes the refactoring actions for C++ code. The action is available when the cursor is in the position described in the Activation column.
Refactoring Action | Description | Activation |
Add Curly Braces | Adds curly braces to an if statement that does not contain a compound statement. For example, rewrites if (a)
b;
as
if (a) {
b;
}
| if |
Move Declaration out of Condition | Moves a declaration out of an if or while condition to simplify the condition. For example, rewrites if (Type name = foo()) {...}
as
Type name = foo;
if (name) {...}
| Name of the introduced variable |
Rewrite Condition Using || | Rewrites the expression according to De Morgan's laws. For example, rewrites: !a && !b
as
!(a || b)
| && |
Rewrite Using operator | Rewrites an expression negating it and using the inverse operator. For example, rewrites:
a op b
as
!(a invop b)
(a op b)
as
!(a invop b)
!(a op b)
as
(a invob b)
| <= < > >= == != |
Split Declaration | Splits a simple declaration into several declarations. For example, rewrites: int *a, b;
as
int *a;
int b;
| Type name or variable name |
Split if Statement | Splits an if statement into several statements. For example, rewrites: if (something && something_else) {
}
as
if (something) {
if (something_else) {
}
}
and
if (something || something_else)
x;
with
if (something)
x;
else if (something_else)
x;
| && || |
Swap Operands | Rewrites an expression in the inverse order using the inverse operator. For example, rewrites: a op b
as
b flipop a
| <= < > >= == != && || |
Convert to Decimal | Converts an integer literal to decimal representation | Numeric literal |
Convert to Hexadecimal | Converts an integer literal to hexadecimal representation | Numeric literal |
Convert to Octal | Converts an integer literal to octal representation | Numeric literal |
Convert to Objective-C String Literal | Converts a string literal to an Objective-C string literal if the file type is Objective-C(++). For example, rewrites the following strings "abcd"
QLatin1String("abcd")
QLatin1Literal("abcd")
as
@"abcd"
| String literal |
Enclose in QLatin1Char(...) | Sets the encoding for a character to Latin-1, unless the character is already enclosed in QLatin1Char, QT_TRANSLATE_NOOP, tr, trUtf8, QLatin1Literal, or QLatin1String. For example, rewrites 'a'
as
QLatin1Char('a')
| String literal |
Enclose in QLatin1String(...) | Sets the encoding for a string to Latin-1, unless the string is already enclosed in QLatin1Char, QT_TRANSLATE_NOOP, tr, trUtf8, QLatin1Literal, or QLatin1String. For example, rewrites "abcd"
as
QLatin1String("abcd")
| String literal |
Mark as Translatable | Marks a string translatable. For example, rewrites "abcd" with one of the following options, depending on which of them is available: tr("abcd")
QCoreApplication::translate("CONTEXT", "abcd")
QT_TRANSLATE_NOOP("GLOBAL", "abcd")
| String literal |
#include Header File | Adds the matching #include statement for a forward-declared class or struct | Forward-declared class or struct |
Add Definition in 'filename' | Inserts a definition stub for a member function declaration in the implementation file | Method name |
Add 'Function' Declaration | Inserts the member function declaration that matches the member function definition into the class declaration. The function can be public, protected, private, public slot, protected slot, or private slot. | Method name |
Add Local Declaration | Adds the type of an assignee, if the type of the right-hand side of the assignment is known. For example, rewrites a = foo();
as
Type a = foo();
where Type is the return type of foo()
| Assignee |
Convert to Camel Case... | Converts a symbol name to camel case, where elements of the name are joined without delimiter characters and the initial character of each element is capitalized. For example, rewrites an_example_symbol as anExampleSymbol and AN_EXAMPLE_SYMBOL as AnExampleSymbol | Identifier |
Complete Switch Statement | Adds all possible cases to a switch statement of the type enum | Switch |
Generate Missing Q_PROPERTY Members... | Adds missing members to a Q_PROPERTY:
- read method
- write method, if there is a WRITE
- on...Changed signal, if there is a NOTIFY
- data member with the name m_<propertyName>
| Q_PROPERTY |
Refactoring QML Code
You can apply the following types of refactoring actions to QML code:
- Rename IDs
- Split initializers
- Move a QML element into a separate file to reuse it in other .qml files
The following table summarizes the refactoring actions for QML code. The action is available when the cursor is in the position described in the Activation column.
Refactoring Action | Description | Activation |
Move Component into 'filename.qml' | Moves a QML element into a separate file | Element name |
Rename id | Renames all instances of an element ID in the currently open file | Element ID |
Split Initializer | Reformats a one-line element into a multi-line element. For example, rewrites Item { x: 10; y: 20; width: 10 }
as
Item {
x: 10;
y: 20;
width: 10
}
| Element property |
|