QUndoView Class
The QUndoView class displays the contents of a QUndoStack. More...
#include <QUndoView> Inherits: QListView.
This class was introduced in Qt 4.2.
Public Types
enum | DragDropMode { NoDragDrop, DragOnly, DropOnly, DragDrop, InternalMove } |
enum | EditTrigger { NoEditTriggers, CurrentChanged, DoubleClicked, SelectedClicked, ..., AllEditTriggers } |
flags | EditTriggers |
enum | PaintDeviceMetric { PdmWidth, PdmHeight, PdmWidthMM, PdmHeightMM, ..., PdmPhysicalDpiY } |
enum | ScrollHint { EnsureVisible, PositionAtTop, PositionAtBottom, PositionAtCenter } |
enum | ScrollMode { ScrollPerItem, ScrollPerPixel } |
enum | SelectionBehavior { SelectItems, SelectRows, SelectColumns } |
enum | SelectionMode { SingleSelection, ContiguousSelection, ExtendedSelection, MultiSelection, NoSelection } |
Properties
Public Functions
Public Slots
- 19 public slots inherited from QWidget
- 1 public slot inherited from QObject
Signals
Protected Types
enum | CursorAction { MoveUp, MoveDown, MoveLeft, MoveRight, ..., MovePrevious } |
enum | DropIndicatorPosition { OnItem, AboveItem, BelowItem, OnViewport } |
enum | State { NoState, DraggingState, DragSelectingState, EditingState, ..., AnimatingState } |
Protected Functions
- 26 protected functions inherited from QListView
- 18 protected functions inherited from QAbstractScrollArea
- 3 protected functions inherited from QFrame
- 37 protected functions inherited from QWidget
- 9 protected functions inherited from QObject
Protected Slots
virtual void | closeEditor(QWidget * editor, QAbstractItemDelegate::EndEditHint hint) |
virtual void | commitData(QWidget * editor) |
virtual void | currentChanged(const QModelIndex & current, const QModelIndex & previous) |
virtual void | dataChanged(const QModelIndex & topLeft, const QModelIndex & bottomRight, const QSet<int> & roles = QSet<int> ()) |
virtual void | editorDestroyed(QObject * editor) |
virtual void | rowsAboutToBeRemoved(const QModelIndex & parent, int start, int end) |
virtual void | rowsInserted(const QModelIndex & parent, int start, int end) |
virtual void | selectionChanged(const QItemSelection & selected, const QItemSelection & deselected) |
virtual void | updateGeometries() |
- 1 protected slot inherited from QWidget
Additional Inherited Members
- 4 static public members inherited from QWidget
- 11 static public members inherited from QObject
Detailed Description
The QUndoView class displays the contents of a QUndoStack.
QUndoView is a QListView which displays the list of commands pushed on an undo stack. The most recently executed command is always selected. Selecting a different command results in a call to QUndoStack::setIndex(), rolling the state of the document backwards or forward to the new command.
The stack can be set explicitly with setStack(). Alternatively, a QUndoGroup object can be set with setGroup(). The view will then update itself automatically whenever the active stack of the group changes.
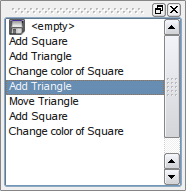
Property Documentation
cleanIcon : QIcon
This property holds the icon used to represent the clean state.
A stack may have a clean state set with QUndoStack::setClean(). This is usually the state of the document at the point it was saved. QUndoView can display an icon in the list of commands to show the clean state. If this property is a null icon, no icon is shown. The default value is the null icon.
Access functions:
QIcon | cleanIcon() const |
void | setCleanIcon(const QIcon & icon) |
This property holds the label used for the empty state.
The empty label is the topmost element in the list of commands, which represents the state of the document before any commands were pushed on the stack. The default is the string "<empty>".
Access functions:
QString | emptyLabel() const |
void | setEmptyLabel(const QString & label) |
Member Function Documentation
QUndoView::QUndoView(QWidget * parent = 0)
Constructs a new view with parent parent.
QUndoView::QUndoView(QUndoStack * stack, QWidget * parent = 0)
Constructs a new view with parent parent and sets the observed stack to stack.
QUndoView::QUndoView(QUndoGroup * group, QWidget * parent = 0)
Constructs a new view with parent parent and sets the observed group to group.
The view will update itself autmiatically whenever the active stack of the group changes.
QUndoView::~QUndoView()
Destroys this view.
QUndoGroup * QUndoView::group() const
Returns the group displayed by this view.
If the view is not looking at group, this function returns 0.
See also setGroup() and setStack().
void QUndoView::setGroup(QUndoGroup * group) [slot]
Sets the group displayed by this view to group. If group is 0, the view will be empty.
The view will update itself autmiatically whenever the active stack of the group changes.
See also group() and setStack().
void QUndoView::setStack(QUndoStack * stack) [slot]
Sets the stack displayed by this view to stack. If stack is 0, the view will be empty.
If the view was previously looking at a QUndoGroup, the group is set to 0.
See also stack() and setGroup().
QUndoStack * QUndoView::stack() const
Returns the stack currently displayed by this view. If the view is looking at a QUndoGroup, this the group's active stack.
See also setStack() and setGroup().
|